- (5.0 Star)
Programming in C#
Certification Training
We provide Online Instructor And Classroom Instructor led Live virtual classroom by certified trainers/ industry professionals
- Get Up to 35% discount
About Course
C# (pronounced “C-sharp”) is an object-oriented programming language from Microsoft that aims to combine the computing power of C++ with the programming ease of Visual Basic. C# is based on C++ and contains features similar to those of Java.C# is designed to work with Microsoft’s .Net platform. Microsoft’s aim is to facilitate the exchange of information and services over the Web, and to enable developers to build highly portable applications. C# simplifies programming through its use of Extensible Markup Language (XML) and Simple Object Access Protocol (SOAP) which allow access to a programming object or method without requiring the programmer to write additional code for each step. Because programmers can build on existing code, rather than repeatedly duplicating it, C# is expected to make it faster and less expensive to get new products and services to market.
Key Features
You will get 100% job Assurance and life time e-placement support
classed taken by globally certified trainers
You will get 3 year Dedicated placement support
Courses are globally recognized & accredited
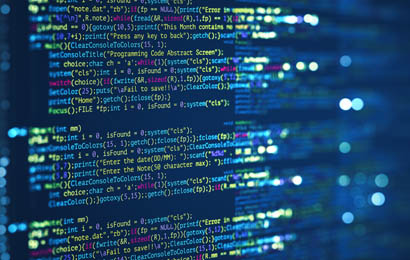
Course Type:
Certification Training
Live virtual classroom:
7,199/-
8,999/-
Regular classroom:
7,199/-
8,999/-
- Duration:
60 Hrs
- Enrolled:
65 Learners
- Eligibility:
Fresher 10th/10+2/Graduate with C/ C++ Skill
- 5 Star:
15 Reviews
Programming In C# Course Curriculum
WORKING WITH BASIC TOOLS :
- .NET Executables and the CLR
- A .NET Testbed for C# Programming
- Using Visual Studio
FIRST C# PROGRAMS
- Hello, World
- Namespaces
- Variables and Expressions
- Using C# as a Calculator
- Input/Output in C#
- .NET Framework Class Library
DATA TYPES IN C#
- Data Types
- Integer Types
- Floating Point Types
- Decimal Type
- Characters and Strings
- Boolean Type
- Conversions
- Nullable Types
OPERATORS AND EXPRESSIONS
- Operator Cardinality
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Expressions
- Checked and Unchecked
CONTROL STRUCTURES
- If Tests
- Loops
- Arrays
- Foreach
- More about Control Flow
- Switch
OBJECT-ORIENTED PROGRAMMING
- Objects
- Classes
- Inheritance
- Polymorphism
- Object-Oriented Languages
- Components
CLASSES
- Classes as Structured Data
- Methods
- Constructors and Initialization
- Static Fields and Methods
- Constant and Readonly
MORE ABOUT TYPES
- Overview of Types in C#
- Value Types
- Boxing and Unboxing
- Reference Types
METHODS, PROPERTIES AND OPERATORS
- Methods
- Parameter Passing
- Method Overloading
- Variable-Length Parameter Lists
- Properties
- Operator Overloading
CHARACTERS AND STRINGS
- Characters
- Strings
- String Input
- String Methods
- String Builder Class
- Programming with Strings
ARRAYS AND INDEXERS
- Arrays
- System.Array
- Random Number Generation
- Jagged Arrays
- Rectangular Arrays
- Arrays as Collections
- Indexers
INHERITANCE
- Single Inheritance
- Access Control
- Method Hiding
- Initialization
VIRTUAL METHODS AND POLYMORPHISM
- Virtual Methods and Dynamic Binding
- Method Overriding
- Fragile Base Class Problem
- Polymorphism
- Abstract Classes
- Sealed Classes
- Heterogeneous Collections
FORMATTING AND CONVERSION
- ToString
- Format Strings
- String Formatting Methods
- Type Conversions
- C Net
EXCEPTIONS
- Exception Fundamentals
- Structured Exception Handling
- User-Defined Exception Classes
- Inner Exceptions
INTERFACES
- Interface Fundamentals
- Programming with Interfaces
- Using Interfaces at Runtime
- Resolving Ambiguities
.NET INTERFACES AND COLLECTIONS
- Collections
- IEnumerable and IEnumerator
- Copy Semantics and ICloneable
- Comparing Objects
- Generic Types
- Type-Safe Collections
DELEGATES AND EVENTS
- Delegates
- Anonymous Methods
- Events
INTRODUCTION TO WINDOWS FORMS
- Creating Windows Applications Using Visual Studio
- Partial Classes
- Buttons, Labels and Textboxes
- Handling Events
- Listbox Controls
ADDITIONAL C# FEATURES
- Auto-Implemented Properties
- Implicitly Typed Variables
- Object Initializers
- Collection Initializers
- Anonymous Types
- Partial Methods
- Extension Methods
- Lambda Expressions
- Language-Integrated Query (LINQ)
Sent Us a Message